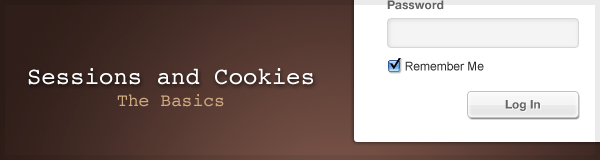
A critical feature in web programming is the ability to seamlessly pass data from one page load to the next. Itâs used most commonly when dealing with user logins, but also for passing error messages, shopping carts, etc.
Storing data across pages using PHP is done with two variables in the global scope, called $_SESSION and $_COOKIE, and although accomplishing the same end goal, the both go about it in very different ways. The purpose of this article is to give a brief look into the differences between cookies and sessions, when itâs better to use one versus the other, and the pros and cons of the two.
The difference is in how each store data. Cookies store data locally in the userâs browser, while sessions store data on the webserver.
Session BasicsSessions are simply server-side cookies each with a corresponding client side cookie that contains only a reference to its server-side counterpart. When a user visits a page, the client sends the reference code to the server, and PHP will then match that reference code to a server-side cookie and load the data in the serverâs cookie into the $_SESSION superglobal.
ProsCookie data is sent to the web server every page load. PHP reads and stores the value into the $_COOKIE superglobal. When a cookie is created, you can give it a lifespan. After that lifespan runs out, it will expire.
ProsThe function definition:
bool setcookie ( string name [, string value [, int expire [, string path [, string domain [, int secure]]]]])
<?php if (!isset($_COOKIE['Ordering'])) { setcookie("Ordering", $_POST['ChangeOrdering'], time() + 31536000); } ?>Using a cookie<?php echo (isset($_COOKIE[âorderingâ])) ? $_COOKIE[âorderingâ] : âcookie value not setâ; ?>Deleting a cookie<?php setcookie(âfavorite_colorâ); ?>Setting a cookie with no value is the same as deleting it. This will not remove the file from the client computer. To do this, you can set the cookie expiration date to a time in the past, and the browser will take care of it.
Sessions in ActionCreating a session<?php session_start(); ?>This must be called near the top of your code before any output. When you call this function, PHP will check to see if the user sent a session cookie. If so, it will load the session data into $_SESSION. If not, it will create a new session file on the server and send the ID back to the client.
Setting a value<?php $_SESSION[âfirst_nameâ] = âBrianâ; ?>Reading a session value<?php echo $_SESSION[âfirst_nameâ]; ?>Removing session data<?php unset($_SESSION[âfirst_nameâ]); ?>Ending a session<?php session_destroy(); ?>The Bottom LineSessions are cookies where the data is stored on the server. Cookies are stored in the users filesystem (typically in their âTemporary Internet Filesâ folder). Both have their advantages, but on any given day, youâll probably find yourself using sessions much more commonly.
PHP DocumentationPowered by WizardRSS | Full Text RSS Feeds